Arrays are fundamental building blocks in PHP, allowing you to store and manage collections of data. But sometimes, you need to remove unwanted elements. Here, we’ll explore five effective ways to achieve this!
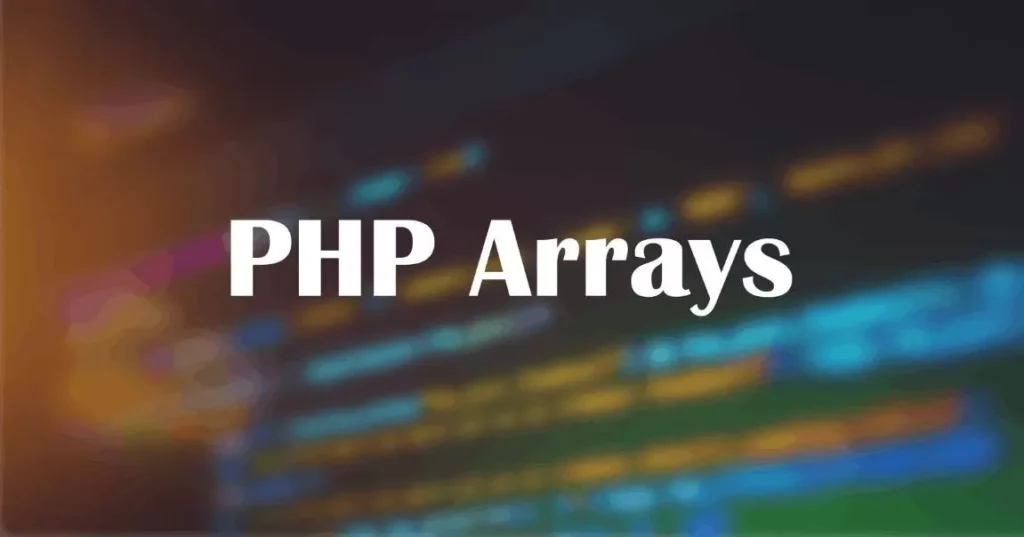
1. unset() – The Simple Deletion
The unset()
function is the most straightforward method for removing an item from an array. It takes the array index or key as its argument and removes the corresponding element. However, keep in mind that:
unset()
doesn’t re-index the array. Gaps will appear where elements were removed.- It works for both numeric and string keys.
Example:
PHP
$fruits = ["apple", "orange", "banana", "pear"];
unset($fruits[1]); // Removes "orange"
print_r($fruits); // Output: Array ( [0] => apple [2] => banana [3] => pear )
2. array_splice() – Precise Removal with Control
For more control over the removal process, array_splice()
is your friend. It allows you to:
- Specify the starting index (
$start
) where removal begins. - Define the number of elements (
$length
) to remove. - Optionally, provide replacement elements that will be inserted at the specified position.
Example:
PHP
$colors = ["red", "green", "blue", "yellow", "purple"];
// Remove "blue" and "yellow"
array_splice($colors, 2, 2);
print_r($colors); // Output: Array ( [0] => red [1] => green [3] => purple )
3. array_shift() & array_pop() – Removing First or Last
These functions are handy for specific scenarios:
array_shift()
: Removes the first element from the array and re-indexes the remaining elements.array_pop()
: Removes the last element from the array.
Example:
PHP
$numbers = [10, 20, 30, 40];
$first_removed = array_shift($numbers); // Removes and stores 10
$last_removed = array_pop($numbers); // Removes and stores 40
print_r($numbers); // Output: Array ( [0] => 20 [1] => 30 )
4. array_diff() & array_diff_key() – Removing Based on Values or Keys
These functions compare your original array with another and return a new array containing only the elements that differ:
array_diff()
: Compares values. Elements with the same value in both arrays are excluded.array_diff_key()
: Compares keys. Elements with the same key in both arrays are excluded.
Example (array_diff):
PHP
$original = [1, 2, 3, 4];
$to_remove = [2, 4];
$filtered_array = array_diff($original, $to_remove);
print_r($filtered_array); // Output: Array ( [0] => 1 [1] => 3 )
5. array_values() – Re-indexing After Removal
If you’ve removed elements using unset()
and want a clean, re-indexed array:
- Use
array_values()
to create a new array containing the remaining elements with continuous numeric indexing.
Example:
PHP
$letters = ["a", "b", "c", "d", "e"];
unset($letters[1]); // Removes "b"
$reindexed_array = array_values($letters);
print_r($reindexed_array); // Output: Array ( [0] => a [1] => c [2] => d [3] => e )
Choosing the Right Method:
The best approach depends on your specific needs:
- For simple removal by index or key:
unset()
- For precise removal with control:
array_splice()
- For removing first/last elements:
array_shift()
orarray_pop()
- For removing based on values/keys:
array_diff()
orarray_diff_key()
- For re-indexing after removal:
array_values()
Remember, understanding these methods empowers you to manage your PHP arrays effectively!