Laravel 11 isn’t just an update; it’s a significant leap forward for web development with Laravel. This release focuses on streamlining workflows, simplifying project management, and introducing powerful new features like Laravel Reverb, a game-changer for real-time applications. Let’s delve deeper into the top new features and explore how they can enhance your development experience.
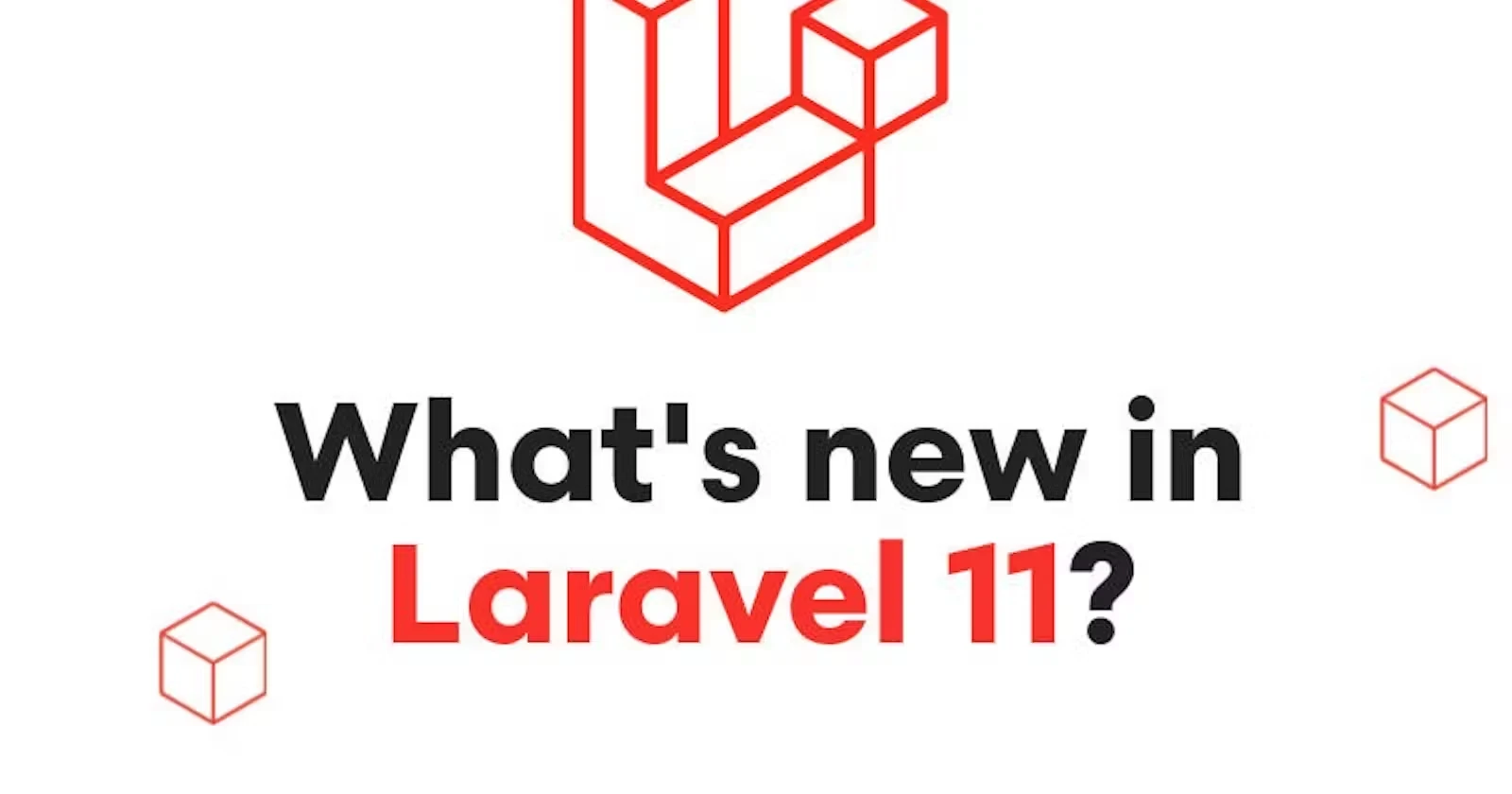
1. Streamlined Application Skeleton: Less Code, More Focus
Say goodbye to sprawling project directories filled with boilerplate code. Laravel 11 introduces a much slimmer application skeleton. Several folders, like app/Console
and app/Exceptions
, have been removed, and configurations are centralized in the bootstrap/app.php
file. This reduces clutter, simplifies project navigation, and allows you to focus on the core functionalities of your application.
Example:
Previously, defining artisan commands required a dedicated folder structure within app/Console
. With Laravel 11, you can define commands directly in the routes/console.php
file. Here’s an example of a simple command that generates a random quote:COPY
// routes/console.php
use Illuminate\Console\Command;
class InspireCommand extends Command
{
protected $signature = 'inspire';
protected $description = 'Display an inspiring quote';
public function handle()
{
$quotes = [
'The best way to predict the future is to create it. - Abraham Lincoln',
'The only limit to our realization of tomorrow will be our doubts of today. - Franklin D. Roosevelt',
];
$this->line($quotes[array_rand($quotes)]);
}
}
2. Pest and SQLite by Default: Faster Testing and Lighter Startups
For new Laravel projects, the framework now embraces Pest as the default testing framework. Pest offers a more enjoyable and streamlined testing experience compared to older options. Additionally, SQLite becomes the default database option. SQLite is a lightweight, in-memory database that’s perfect for rapid development and getting started with your project without the need for complex database configurations.
Example:
Writing unit tests for your models and controllers is essential for ensuring code quality. Here’s a simple Pest test for a User model with validation rules:COPY
// tests/Unit/UserTest.php
use App\Models\User;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Illuminate\Support\Facades\Validator;
it('validates required fields', function () {
$user = User::new();
$validator = Validator::make($user->toArray(), [
'name' => 'required',
'email' => 'required|email',
]);
expect($validator->fails())->toBeTrue();
});
3. Health Check for Proactive Monitoring:
Keeping your application running smoothly is crucial. Laravel 11 introduces a health check endpoint (/up
). This endpoint allows you to monitor vital aspects of your application’s health, such as database connections, cache status, and queue health. With a quick API call, you can identify potential issues before they impact user experience.
Example:
Imagine you have a complex e-commerce application relying heavily on a Redis cache for product data. Using the health check endpoint, you can set up automated scripts or monitoring tools to periodically check the cache status. If the health check detects an issue with the cache connection, you can be alerted and take corrective actions before users encounter problems loading product information.
4. Dumpable Trait: Effortless Debugging with a Single Line
Debugging can be a time-consuming process. Laravel 11 introduces the Dumpable
trait, offering a convenient way to inspect variables and data structures directly within your code. No more complex dd
or var_dump
statements! Simply add the Dumpable
trait to your model or class, and you can use the dump
method to instantly view the contents of any variable.
Example:
Struggling to understand why a specific calculation in your controller isn’t producing the expected result? With the Dumpable
trait, you can easily inspect the values of variables involved in the calculation:COPY
<?php
use Illuminate\Database\Eloquent\Model;
use Illuminate\Contracts\Support\Dumpable;
class Product extends Model implements Dumpable
{
public function calculateDiscountPrice()
{
$discount = 0.1; // Assuming a 10% discount
$price = $this->price;
$discountedPrice = $price * (1 - $discount);
dump(compact('price', 'discount', 'discountedPrice'));
return $discountedPrice;
}
}
5. Laravel Reverb: Real-Time Powerhouse Enters the Stage
Now, let’s explore the most exciting addition to Laravel 11: Laravel Reverb. This revolutionary feature introduces a first-party WebSocket server, enabling real-time communication between your application and users’ browsers. WebSockets establish a persistent two-way connection, perfect for building dynamic and interactive features like:
- Chat applications: Imagine a chat app where users see messages the instant they’re sent, fostering a seamless and engaging conversation experience.
- Live dashboards: Real-time data updates on dashboards empower users to stay informed about critical metrics or changes within your application.
- Collaborative editing: Multiple users can work on a document simultaneously, seeing each other’s edits in real-time, perfect for collaborative writing or design tools.
Benefits of Laravel Reverb:
- Seamless Integration: Built by the Laravel team, Reverb integrates effortlessly with your Laravel application, minimizing configuration overhead.
- Scalability: Reverb is designed to handle a high volume of concurrent connections, ensuring your application can scale efficiently as your user base grows.
- Reduced Server Load: Compared to traditional approaches like polling, WebSockets with Reverb minimize server load by establishing a single connection per user. This translates to improved performance and resource efficiency.
Example: Building a Real-Time Chat App with Laravel Reverb
Let’s illustrate the power of Laravel Reverb with a simple example: a real-time chat application. Here’s a breakdown of the key steps involved:
- Setting Up Laravel Reverb: The installation process for Reverb is straightforward. You’ll need to add the
laravel/reverb
package to your project and configure the WebSocket server within your Laravel application configuration. - Establishing WebSocket Connections: Your application will handle establishing WebSocket connections with user browsers. This typically involves utilizing JavaScript libraries on the client-side to initiate the connection and handle communication with the server.
- Broadcasting Messages: When a user sends a message, your Laravel application will leverage Reverb to broadcast that message to all connected users. Reverb will efficiently handle the real-time delivery of the message to each user’s browser.
- Receiving and Displaying Messages: On the client-side, JavaScript code will listen for incoming messages from the WebSocket connection. Once a message is received, it can be displayed in real-time within the chat interface, providing a seamless and interactive experience for users.
Conclusion
Laravel 11 empowers developers with a range of features that streamline project management, simplify debugging, and introduce the game-changing power of real-time interactions with Laravel Reverb. This comprehensive update paves the way for a more efficient and engaging web development experience, allowing you to build modern applications that cater to the evolving needs of today’s users. So, embrace Laravel 11 and unlock the potential for faster development cycles, enhanced debugging capabilities, and real-time interactions that will elevate your web applications to the next level.